목록전체 글 (134)
Foggy day
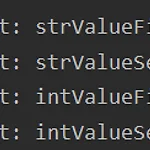
Elvis of Kotlin is equal Optional.getOrElse() of java. you can set the default value of variable by using { ?: } class KotlinPlayGroundActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_kotlin_play_ground) var str: String? = null var strValueFirst = str ?: "It is null" println("strValueFirst : ..
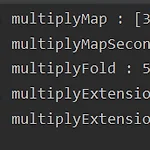
class KotlinPlayGroundActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_kotlin_play_ground) val lists = listOf(1, 2, 3, 4, 5, 6, 7) println("multiplyMap : ${multiplyMap(lists)} ") println("multiplyMapSecond : ${multiplyMapSecond(lists)}") println("multiplyFold : ${multiplyFold(lists)} ") print..
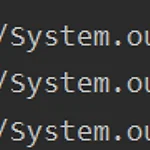
Fold function have initial value. initial is 1 in this example. so, result of calFold is 25. But reduce function don't have initial value. so, result of calRecude is 24, because value of List position zero is 0. class KotlinPlayGroundActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_kotlin_pla..
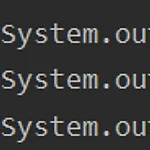
class KotlinPlayGroundActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_kotlin_play_ground) println("calculate : ${calculate(3, 4)}") println("calculate2 : ${calculate2(5, 10)}") println("calculate3 : ${calculate3(20, 41)}") } fun calculate(a: Int, b: Int): Int { return a + b } fun calculate2(..
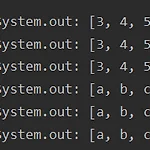
In Kotlin, there are two types immutable and mutable. The first is the immutable list. If you use +(plus) operator, plus value would be added to the list. The important thing is the fact that +(plus) operator create a new list. class KotlinPlayGroundActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.act..
If you want to create Singleton in Kotlin, just add object instead of class. Singleton can have only one instance. object(singleton) don't have constructor. object MyWindowAdapter : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) } }
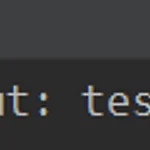
Kotlin don't have static. But it have companion object. class KotlinPlayGroundActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_kotlin_play_ground) Person.create("companion object text") } data class Person(val name: String, val registered: Instant = Instant.now()) { companion object { fun cre..
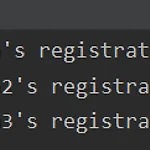
If you use destructing, you can access to object properties much easier. class KotlinPlayGroundActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_kotlin_play_ground) val persons = listOf(Person("jinhan"), Person("jinhan2"), Person("jinhan3")) show(persons) } data class Person(val name: String, ..
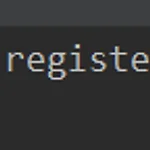
If you declare a value of variable in constructor, you don't need add parameter when you create class. class KotlinPlayGroundActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_kotlin_play_ground) val person = Person("jinhan") println("person name : ${person.name}, registered : ${person.register..
Add open in front of class to extend class in kotlin. class KotlinPlayGroundActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_kotlin_play_ground) val people = People("jinhan", 30) println("name : ${people.name}, age : ${people.age}") } class People(name: String, age: Int) : Person(name, age) {..